Integrating Python with Selenium WebDriver for Web Testing
python automation testing
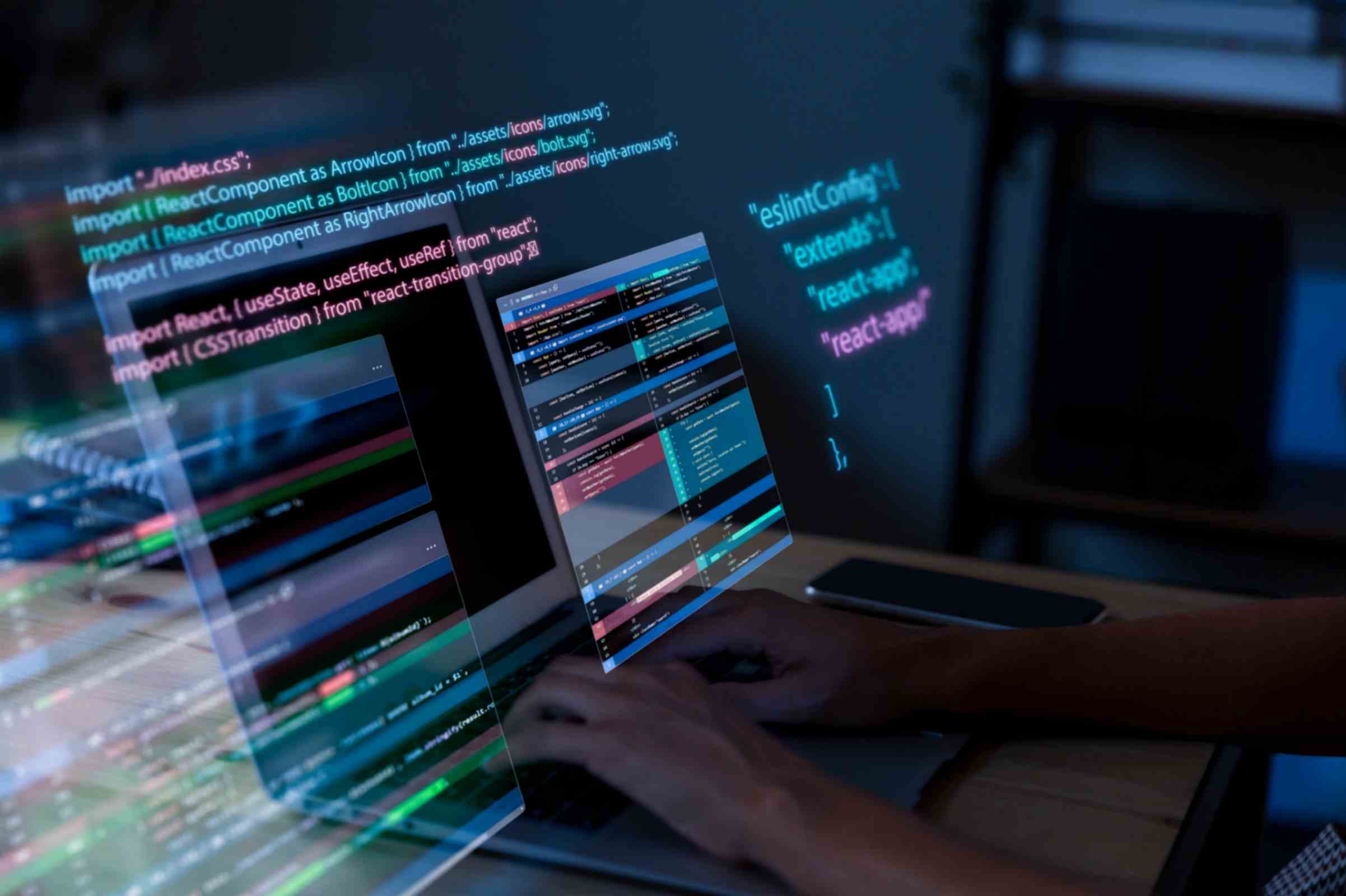
Integrating Python with Selenium WebDriver for Web Testing
In today's digital age, ensuring the quality and functionality of web applications is paramount. One of the most effective ways to achieve this is through automated testing, which not only saves time but also enhances accuracy and efficiency. Python, a versatile and powerful programming language, is widely used in automation testing, particularly in conjunction with Selenium WebDriver, a popular tool for web application testing. This article explores the integration of selenium webdriver python for python for automation testing , offering insights into its benefits, implementation, and best practices.
Table of Contents
Sr# |
Headings |
1 |
Introduction |
2 |
Overview of Python for Automation Testing |
3 |
Introduction to Selenium WebDriver |
4 |
Installing Selenium WebDriver with Python |
5 |
Basic Selenium WebDriver Commands in Python |
6 |
Advanced Selenium WebDriver Commands in Python |
7 |
Handling Dynamic Web Elements with Selenium |
8 |
Implementing Page Object Model (POM) Pattern |
9 |
Best Practices for Integrating Python with Selenium WebDriver |
10 |
Conclusion |
11 |
FAQs |
1. Introduction
Automation with Python is crucial for ensuring the reliability and efficiency of web applications. Python's simplicity and readability make it an ideal choice for writing automated test scripts. When combined with Selenium WebDriver, Python becomes a powerful tool for automation python web testing tasks.
2. Overview of Python for Automation Testing
Python is a popular programming language known for its simplicity and readability. It offers a rich set of libraries and frameworks that make it ideal for automation testing. Python's versatility allows testers to write clear and concise test scripts, reducing the time and effort required for testing.
3. Introduction to Selenium WebDriver
python selenium tutorial WebDriver is a powerful tool for automating web browser interaction. It allows testers to simulate user actions such as clicking buttons, entering text, and navigating between pages. Selenium WebDriver supports multiple programming languages, including Python, making it a popular choice for web testing.
4. Installing Selenium WebDriver with Python
To use Selenium WebDriver with Python, you first need to install the Selenium WebDriver library using pip, Python's package manager. You can install it by running the following command:
bash
Copy code
pip install selenium
5. Basic Selenium WebDriver Commands in Python
Once python automation testing Selenium WebDriver is installed, you can start writing test scripts in Python. Here are some basic commands to get you started:
- Launching a browser:
python
Copy code
from selenium import webdriver
driver = webdriver.Chrome()
- Navigating to a URL:
python
Copy code
driver.get("https://www.example.com")
- Finding an element by ID and clicking it:
python
Copy code
element = driver.find_element_by_id("myButton")
element.click()
6. Advanced Selenium WebDriver Commands in Python
Selenium WebDriver offers a wide range of commands for interacting with web elements. Some advanced commands include:
- Finding an element by class name:
python
Copy code
element = driver.find_element_by_class_name("myClass")
- Executing JavaScript code:
python
Copy code
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
7. Handling Dynamic Web Elements with Selenium
Web pages often contain dynamic elements that change based on user interactions or external factors. Selenium WebDriver provides several methods for handling dynamic elements, such as:
- Implicit and Explicit Waits:
python
Copy code
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "myDynamicElement"))
)
8. Implementing Page Object Model (POM) Pattern
The Page Object Model (POM) is a design pattern used to represent web pages as objects in automated tests. This pattern helps in organizing test code and improving code reusability. Here's how you can implement POM with Selenium WebDriver in Python:
python
Copy code
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_textbox = "username"
self.password_textbox = "password"
self.login_button = "login"
def login(self, username, password):
self.driver.find_element_by_id(self.username_textbox).send_keys(username)
self.driver.find_element_by_id(self.password_textbox).send_keys(password)
self.driver.find_element_by_id(self.login_button).click()
9. Best Practices for Integrating Python with Selenium WebDriver
- Use meaningful variable names and comments to improve code readability.
- Modularize test scripts to promote code reusability.
- Use version control systems like Git to manage test scripts and collaborate with team members.
- Regularly update Selenium WebDriver and Python libraries to leverage the latest features and enhancements.
10. Conclusion
Integrating Python with Selenium WebDriver offers a powerful solution for automating web testing tasks. Python's simplicity and readability, combined with Selenium WebDriver's robust features, make it an ideal choice for testers looking to enhance their testing capabilities. By following best practices and leveraging advanced Selenium WebDriver commands, testers can streamline their testing processes and improve the overall quality of web applications.
11. FAQs
Q1: What is Selenium WebDriver?
A1: Selenium WebDriver is a tool for automating web browser interactions. It allows testers to simulate user actions such as clicking buttons, entering text, and navigating between pages.
Q2: Why use Python for automation testing?
A2: Python is known for its simplicity and readability, making it easy to write and maintain test scripts. It also offers a rich set of libraries and frameworks for Automation Testing with Python .
Q3: How do I install Selenium WebDriver with Python?
A3: You can install Selenium WebDriver with Python using pip, Python's package manager. Simply run the command pip install selenium in your terminal or command prompt.
Q4: What is the Page Object Model (POM) pattern?
A4: The Page Object Model (POM) is a design pattern used in automation testing to represent web pages as objects. This pattern helps in organizing test code and improving code reusability.
Q5: How can I handle dynamic web elements with Selenium WebDriver?
A5: Selenium WebDriver provides several methods for handling dynamic elements, such as implicit and explicit waits. These methods allow you to wait for elements to appear or become clickable before interacting with them.
By integrating Python with Selenium WebDriver, testers can streamline their testing processes, improve test coverage, and ensure the quality and reliability of web applications. Whether you're a seasoned tester or just starting with automation testing, Python and Selenium WebDriver offer a powerful combination for web testing success.